第1章 绪论
第2章 线性表
第3章 栈、队列和数组
第4章 串
第5章 树与二叉树
第6章 图
第7章 查找
为什么要记录这个,事实上,不记录,我电脑的文件里面肯定找不到,然后就忘记了。就像笔记一样,好记性不如烂笔头。
顺序查找和二分查找
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| #include <bits/stdc++.h> #include <deque> #include <iostream> #include <stdio.h> #include <stdlib.h> #include <vector> using namespace std; typedef int ElemType; typedef struct { ElemType *elem; int TableLen; } SSTable;
int Search_Seq(SSTable ST, ElemType key) { int i; for (i = 0; i < ST.TableLen && key != ST.elem[i]; i++) ; return i == ST.TableLen ? -1 : i; }
int Search_Seq1(SSTable ST, ElemType key) { ST.elem[0] = key; int i; for (i = ST.TableLen; key != ST.elem[i]; i--) ; return i; }
int Binary_Search(SSTable L, ElemType key) { int low = 0, high = L.TableLen - 1, mid; while (low <= high) { mid = low + (high - low >> 1); if (key > L.elem[mid]) low = mid + 1; else if (key < L .elem[mid]) high = mid - 1; else return mid; } return -1; } int main() { SSTable t; t.TableLen = 12; t.elem = (ElemType *) calloc(t.TableLen, sizeof(ElemType)); t.elem[11] = 33; t.elem[1] = 10; t.elem[2] = 38; t.elem[3] = 36; t.elem[4] = 325; t.elem[5] = 311; t.elem[6] = 3636; t.elem[7] = 355; t.elem[8] = 43; t.elem[9] = 3543; t.elem[10] = 322; cout << Search_Seq1(t, 43) << endl; return 0; }
|
分块查找(顺序索引查找)
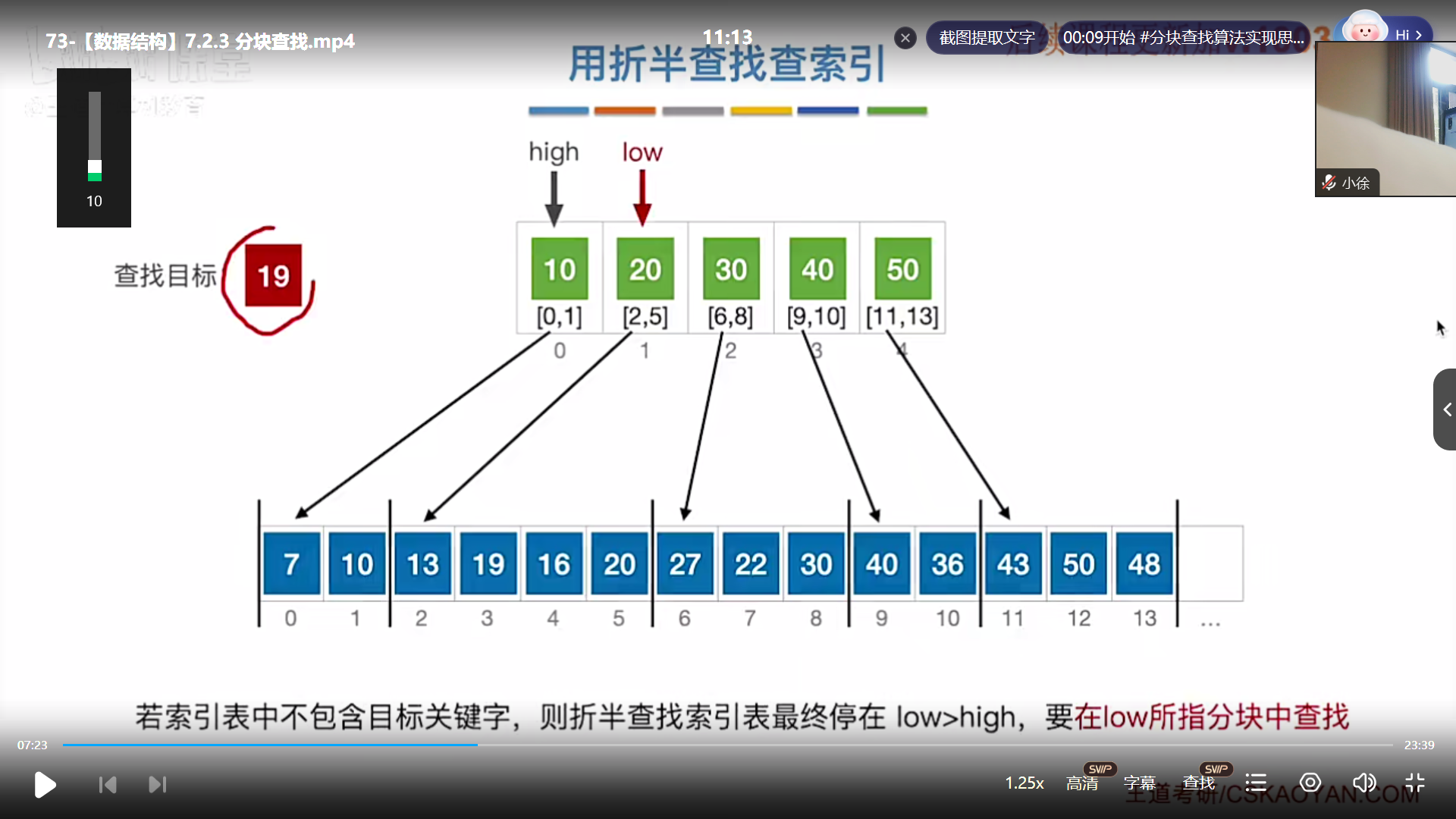
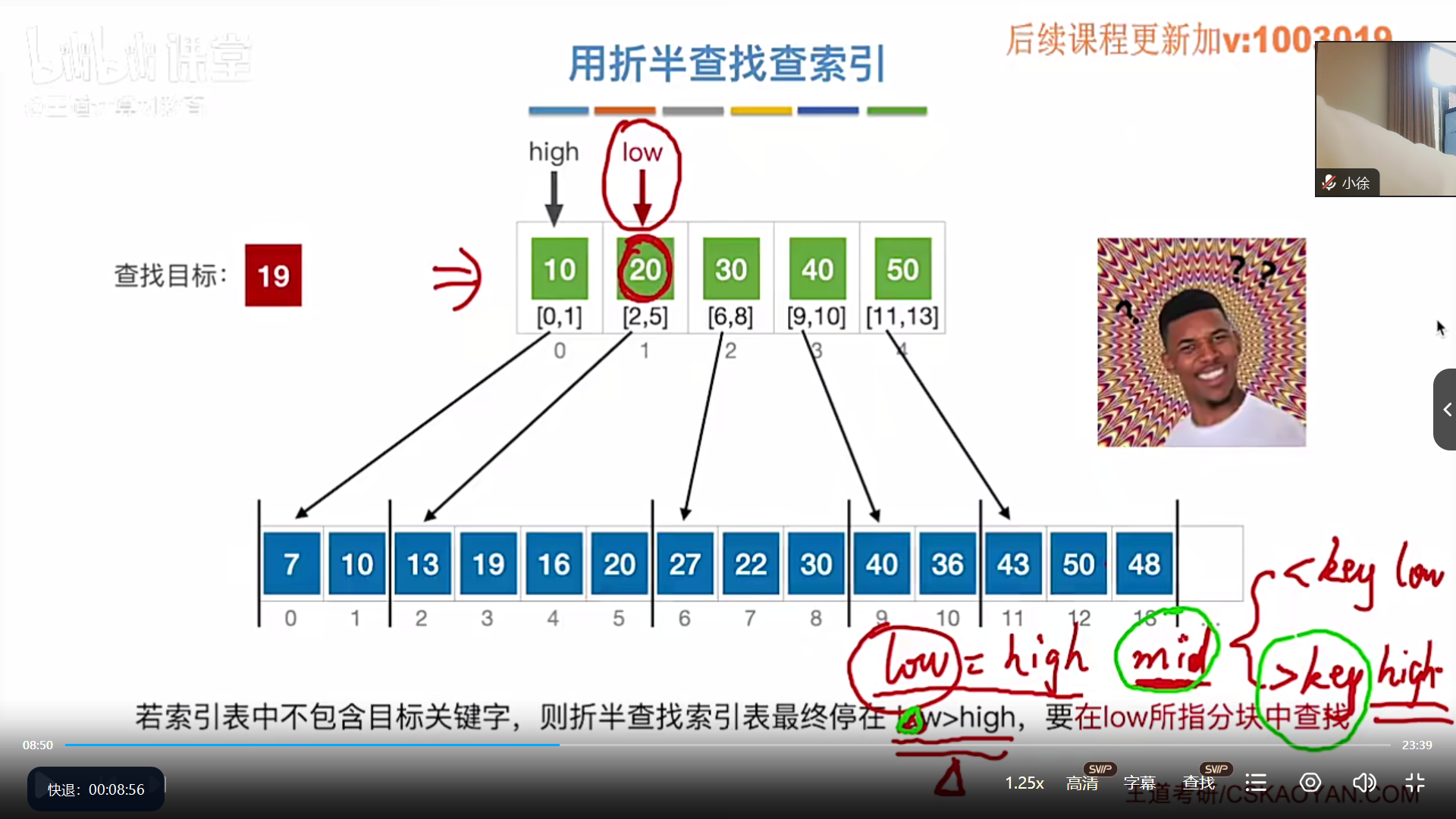
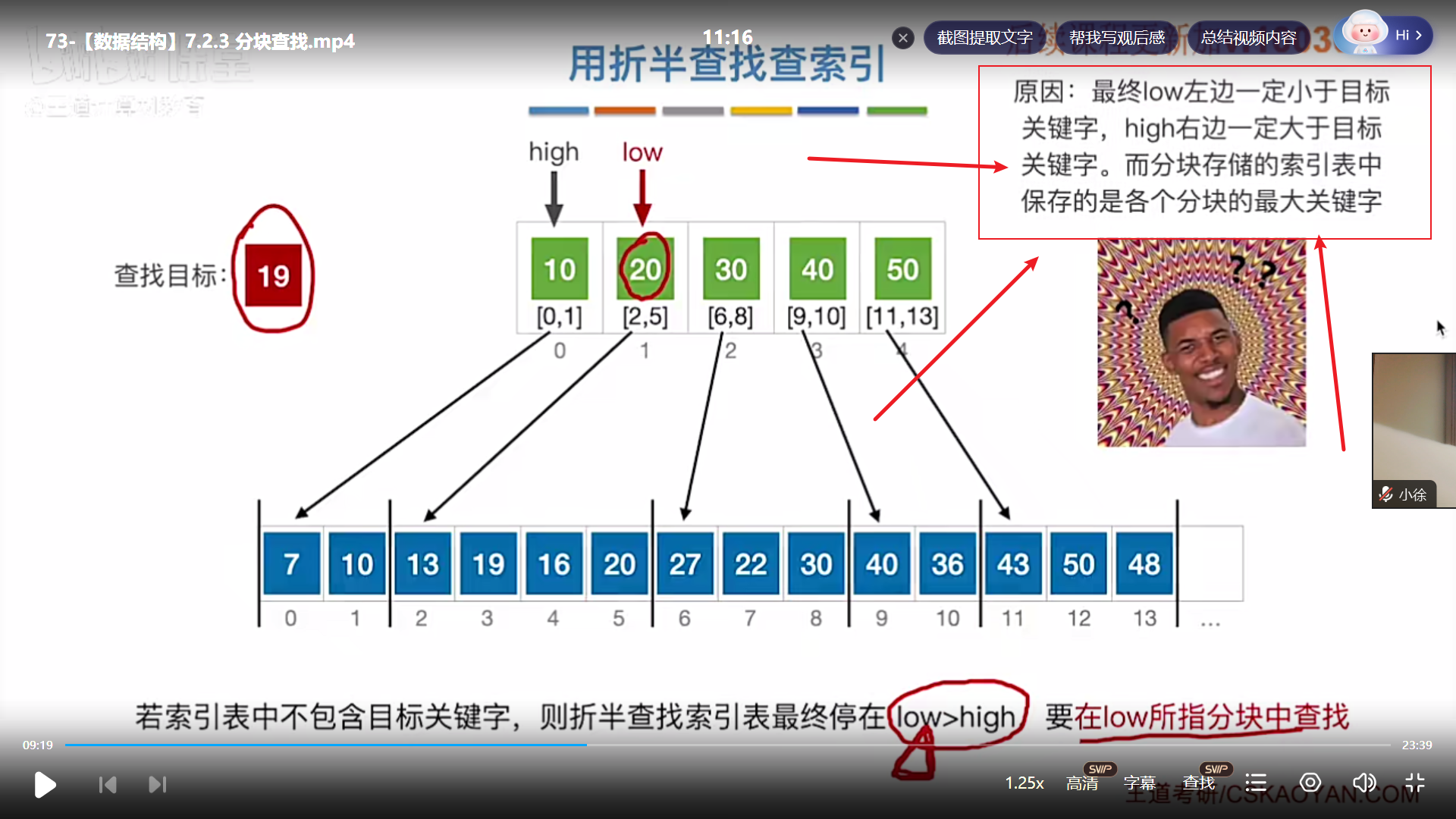
代码王道里面没有,
王道代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| #include <bits/stdc++.h> #include <deque> #include <iostream> #include <stdio.h> #include <stdlib.h> #include <vector> using namespace std; typedef int ElemType;
typedef struct{ ElemType maxValue; int low, high; }Index;
ElemType List[100]; int main() { return 0; }
|
下面是网上找的
【查找系列_分块查找(索引顺序查找)[关键在于确定索引项位置]】 https://www.bilibili.com/video/BV1N24y1Z7eC/?share_source=copy_web&vd_source=82180e49f17daecf14bb6f246fc29cd0
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| #include <bits/stdc++.h> #include <deque> #include <iostream> #include <stdio.h> #include <stdlib.h> #include <vector> using namespace std; typedef struct IndexElem { int maxKey; int low, high; } IndexElem; #define maxSize 5
int searchIndexPos(IndexElem indexTable[], int target) { if (indexTable[maxSize - 1].maxKey < target) return -1;
int low = 0, high = maxSize - 1; while (low <= high) { int mid = low + (high - low >> 1); if (target < indexTable[mid].maxKey) high = mid - 1; else if (target > indexTable[mid].maxKey) low = mid + 1; else return mid; } return low; }
int blockSearch(IndexElem indexTable[], int arr[], int target) { int pos = searchIndexPos(indexTable, target); if (pos < 0) return -1; int low = indexTable[pos].low; int high = indexTable[pos].high; for (int i = low; i <= high; i++) { if (arr[i] == target) return i; } return -1; } int main() { int arr[] = {2, 1, 0, 3, 5, 4, 7, 6, 8, 9, 10, 11, 15, 18, 20}; IndexElem indexTable[maxSize] = { {2, 0, 2}, { 5, 3, 5, }, {8, 6, 8}, {11, 9, 11}, {20, 12, 14}}; printf("%d", blockSearch(indexTable, arr, 12));
return 0; }
|
二叉排序树
C++49的BST的插入
结构体定义
1 2 3 4 5 6 7 8 9 10 11 12 13
| typedef struct tree_node { K key; struct tree_node* left; struct tree_node* right;
}TreeNode;
typedef struct { TreeNode* root; }BST;
|
插入实现,其实这个tree就是二级指针
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| void bst_insert(BST* tree, K key) {
TreeNode* parent = NULL; TreeNode* curr = tree->root; int cmp; while (curr) { cmp = key - curr->key; if (cmp < 0) { parent = curr; curr = curr->left; } else if (cmp > 0) { parent = curr; curr = curr->right; } else { return; } } TreeNode* newNode = calloc(1, sizeof(TreeNode)); if (!newNode) { fprintf(stderr, "malloc failed in bst_insert\n"); exit(1); } newNode->key = key;
if (parent == NULL) { tree->root = newNode; } else if (cmp < 0) parent->left = newNode; else parent->right = newNode; return; }
|
王道代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| #include <bits/stdc++.h> #include <deque> #include <iostream> #include <stdio.h> #include <stdlib.h> #include <vector> using namespace std; #define SIZE(a) (sizeof(a) / sizeof(a[0]))
typedef struct BSTNode { int key; struct BSTNode *lchild, *rchild; } BSTNode, *BSTree;
BSTNode *BST_Search(BSTree T, int key) { while (T != NULL && key != T->key) { if (key < T->key) T = T->lchild; else T = T->rchild; } return T; }
BSTNode *BSTSearch(BSTree T, int key) { if (T == NULL) return NULL; if (key < T->key) BSTSearch(T->lchild, key); else if (key > T->key) BSTSearch(T->rchild, key); else return T; }
int BST_Insert(BSTree &T, int k) { if (T == NULL) { T = (BSTNode *) calloc(1, sizeof(BSTNode)); T->key = k; return 1; } else if (k < T->key) BST_Insert(T->lchild, k); else if (k > T->key) BST_Insert(T->rchild, k); else return 0; }
int BSTInsert(BSTree &T, int k) { } void Create_BST(BSTree &T, int str[], int n) { T = NULL; int i = 0; while (i < n) { BST_Insert(T, str[i]); i++; } } int main() { BSTree T; int str[] = {50, 66, 60, 26, 21, 30, 70, 68}; int size = SIZE(str); printf("%d\n", size); Create_BST(T, str, size); return 0; }
|
王道代码没有删除操作,网上找的一个讲的封神的老师的视频
【懒猫老师-数据结构-(58)二叉排序树的删除(二叉查找树)】 https://www.bilibili.com/video/BV1EK4y1e7UY/?share_source=copy_web&vd_source=82180e49f17daecf14bb6f246fc29cd0
完成了他这一p作业一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169
| #include <bits/stdc++.h> #include <deque> #include <iostream> #include <stdio.h> #include <stdlib.h> #include <vector> using namespace std; #define SIZE(a) (sizeof(a) / sizeof(a[0]))
typedef struct BSTNode { int key; struct BSTNode *lchild, *rchild; } BSTNode, *BSTree;
BSTNode *BST_Search(BSTree T, int key) { while (T != NULL && key != T->key) { if (key < T->key) T = T->lchild; else T = T->rchild; } return T; }
BSTNode *BSTSearch(BSTree T, int key) { if (T == NULL) return NULL; if (key < T->key) BSTSearch(T->lchild, key); else if (key > T->key) BSTSearch(T->rchild, key); else return T; }
int BST_Insert(BSTree &T, int k) { if (T == NULL) { T = (BSTNode *) calloc(1, sizeof(BSTNode)); T->key = k; return 1; } else if (k < T->key) BST_Insert(T->lchild, k); else if (k > T->key) BST_Insert(T->rchild, k); else return 0; }
int BSTInsert(BSTree &T, int k) { }
void deleteNode(BSTree &T) { if (T->lchild == NULL && T->rchild == NULL) { BSTNode *p = T;
T = NULL; delete (p); } else if (T->rchild == NULL) { BSTNode *p = T; T = T->lchild; delete (p); } else if (T->lchild == NULL) { BSTNode *p = T; T = T->rchild; delete (p); } else { BSTNode *parent = T; BSTNode *pre = T->lchild; while (pre->rchild) { parent = pre; pre = pre->rchild; } T->key = pre->key;
if (parent != T) parent->rchild = pre->lchild; else parent->lchild = pre->lchild; delete (pre); } }
bool deleteBST(BSTree &T, int key) { if (T == NULL) return false; else if (T->key == key) { deleteNode(T); return true; } else if (key < T->key) deleteBST(T->lchild, key); else deleteBST(T->rchild, key); } void Create_BST(BSTree &T, int str[], int n) { T = NULL; int i = 0; while (i < n) { BST_Insert(T, str[i]); i++; } } void visit(BSTree T) { printf("%4d", T->key); } void inOrder(BSTree T) { if (T == NULL) return; inOrder(T->lchild); visit(T); inOrder(T->rchild); } int main() { BSTree T; int str[] = {38, 12, 34, 56, 13, 6, 98, 3, 17, 40, 78}; int size = SIZE(str); printf("%d\n", size); Create_BST(T, str, size); inOrder(T); printf("\n");
bool result = deleteBST(T, 999); inOrder(T); if (result) printf("\nTrue"); else printf("\nFalse");
return 0; }
|
完成了他这一p作业二
在不增加结点情况下,将二叉排序树转换成有序双向链表
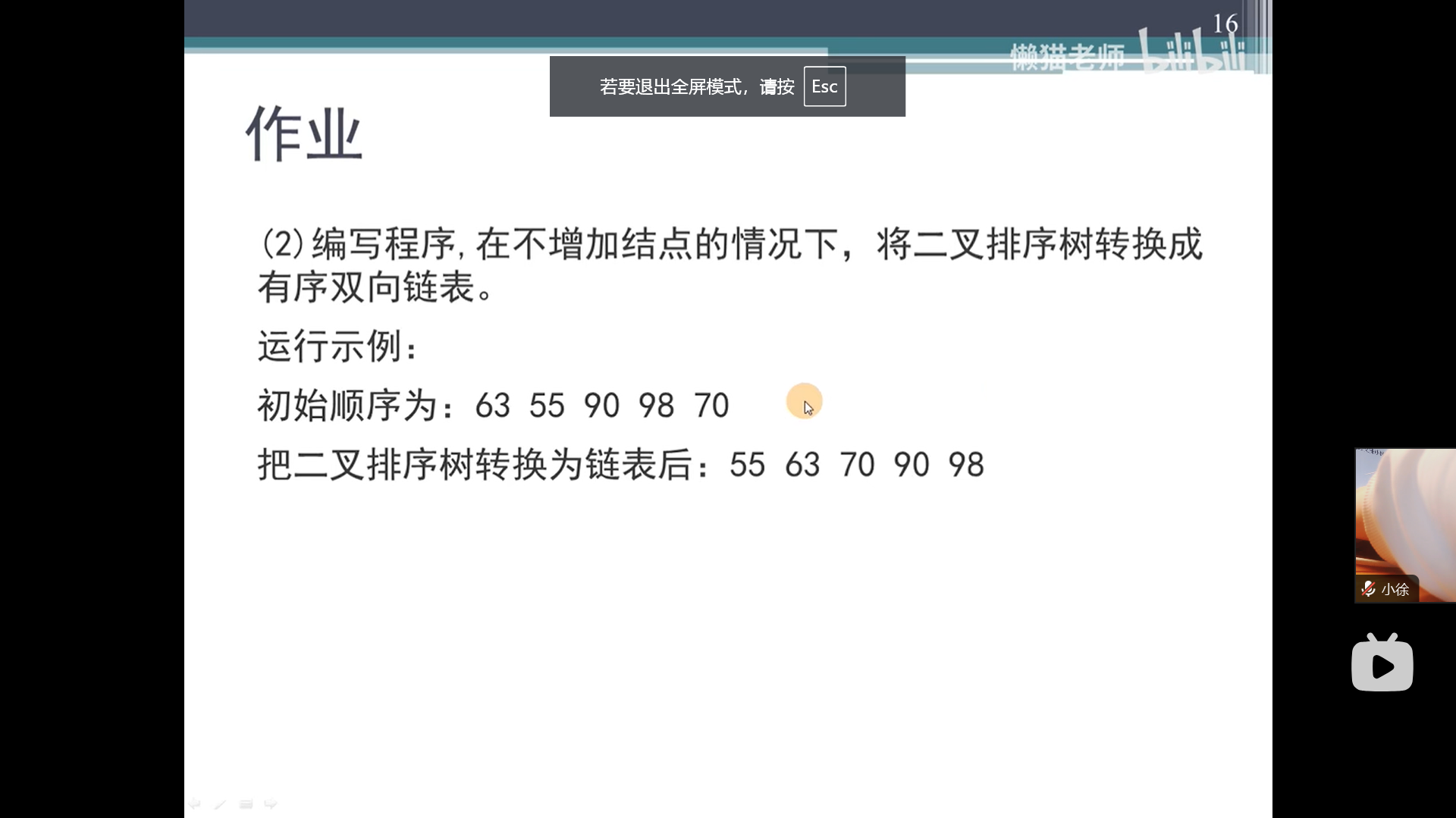
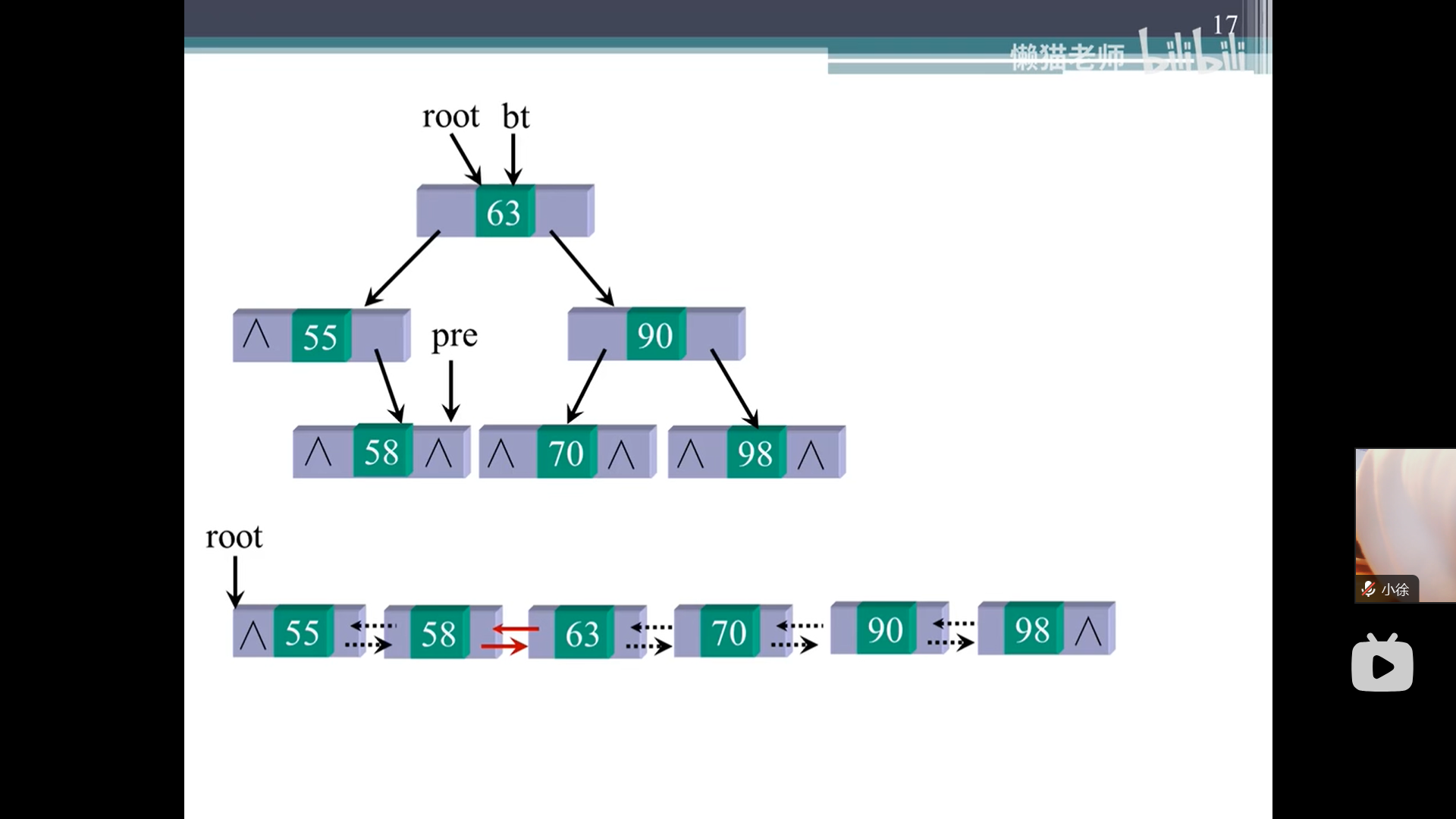
题目要求不能创建任何新的结点,只需要调整指针的指向,那么就意味着可直接利用二叉树的结点,通过变更结点的左右孩子的指向,来生成双链表的逻辑关系。问题就变成了:
找当前root
结点的左孩子最大的结点left_max
,和找当前root
结点的右孩子最大的结点right_max
然后变更各自的指向,依次递归,然后变更指针指向关系:
1 2 3 4
| left_max->rchild= root; root->lchild = left_max; right_max->lchild = root; root->rchild = right_max;
|
————————————————
解题算法
第8章 排序